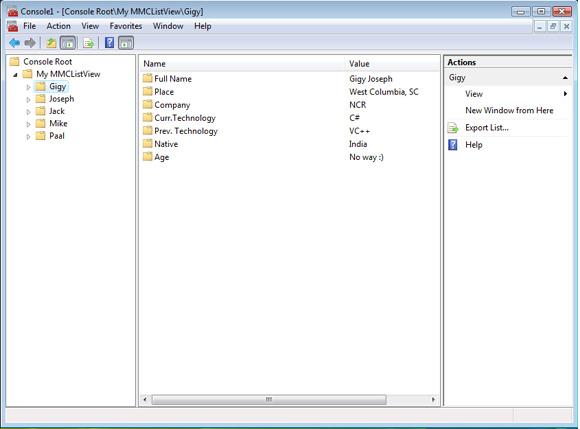
Introduction
The MMC list view shows a list of result nodes in the results pane. This is the most commonly used view that is particularly suited to snap-ins that are designed to manage a dynamic list of items. The MMC list view supports small icons, large icons, lists, and report views. It also supports multi-select and single select operations on items, and a multiple column view with the ability to sort by column.
Using the code
Please make sure that you have gone through the previous articles, if you are a beginner. This article demonstrates how to create a snap-in that uses an MMC list view. I have started by creating a C# class library. Add a reference to Microsoft.ManagementConsole.
Inherit the main class from the SnapIn class

[SnapInSettings("{F98E33A4-1DA5-414c-8576-B3582433F8AB}",
DisplayName = "My Custom MmcListView",
Description = "My Custom MmcListView")]
public class MMCListViewSnapInClass : SnapIn
Add another class which is inheriting from the SnapInInstaller

[RunInstaller(true)]
public class MMCListViewSnapInstallerSupport : SnapInInstaller
{
}
Initializes a new instance of the MMCListViewSnapInClass class. Create a Root Node and set the properties.

// Create a message view for the root node.
MmcListViewDescription lvd = new MmcListViewDescription();
lvd.DisplayName = "My Special MMCListView";
lvd.ViewType = typeof(RootListView);
lvd.Options = MmcListViewOptions.ExcludeScopeNodes;
// Attach the view to the root node.
this.RootNode.ViewDescriptions.Add(lvd);
this.RootNode.ViewDescriptions.DefaultIndex = 0;
In the above code I have created a message view for the root node and attach the view to the root node. The message view is created as RootListView class . This is a new class inherited from MmcListView. The new class looks like this.

using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.ManagementConsole;
using System.Windows.Forms;
namespace MMCListViewSnapIn
{
class RootListView : MmcListView
{
///
/// Initializes a new instance of the RootListView class.
///
public RootListView()
{
}
Since this RootListView class is attached to the Root Node MyMMCListView as MmcListView, this will get loaded on the content pane when Root Node is selected in the MMC tree region.
We can override the OnInitialize to customise the initial view of the content pane.

protected override void OnInitialize(AsyncStatus status)
{
base.OnInitialize(status);
// Your Initialization code can be added here
}
I have added another 5 more MmcListView derived classes which bascailly attached to each child node of the tree. This is the screen shot of the IDE. This may help you sometimes, if you miss something on the go.
Let me explain one of the MmcListView derived class. I am picking MyCustomSelistView class.

using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.ManagementConsole;
using System.Windows.Forms;
namespace MMCListViewSnapIn
{
class MyCusomtSelistView:MmcListView
{
///
/// Initializes a new instance of the MyCusomtSelistView class.
///
public MyCusomtSelistView()
{
}
Override OnInitialize function to set the default setting for the content pane.

protected override void OnInitialize(AsyncStatus status)
{
// this does the default handling
base.OnInitialize(status);
// Create a set of columns for use in the list view
// Define the default column title
this.Columns[0].Title = "Name";
this.Columns[0].SetWidth(300);
// Add detail column
// another way to add the column
this.Columns.Add(new MmcListViewColumn("Value", 500));
// Set to show all columns on the UI
this.Mode = MmcListViewMode.Report; // default (set for clarity)
// Set to show refresh as an option
this.SelectionData.EnabledStandardVerbs = StandardVerbs.Refresh;
// Load the list with values
Refresh();
}
///
/// Loads the list view with data.
///
public void Refresh()
{
// Clear existing information.
this.ResultNodes.Clear();
// Use fictitious data to populate the lists.
string[][] details = { new string[] {"Full Name ", "Gigy Joseph"},
new string[] {"Place", "West Columbia, SC"},
new string[] {"Company", "NCR"},
new string[] {"Curr.Technology", "C#"},
new string[] {"Prev. Technology", "VC++"},
new string[] {"Native", "India"},
new string[] {"Age", "No way :) "},
};
// Populate the list.
foreach (string[] detail in details)
{
ResultNode node = new ResultNode();
node.DisplayName = detail[0];
node.SubItemDisplayNames.Add(detail[1]);
this.ResultNodes.Add(node);
}
}
I have added two columns as Name and Value.Show all columns on the UI by setting Mode as MmcListViewMode.Report. The Refresh function loads data to the list view.

// If there are no selected nodes, then this method simply clears
// the selection data; otherwise, it populates the selection data. It also
// adds a new action that shows the selected items to the actions pane.
protected override void OnSelectionChanged(SyncStatus status)
{
if (this.SelectedNodes.Count == 0)
{
this.SelectionData.Clear();
}
else
{
this.SelectionData.Update(GetDetails(), this.SelectedNodes.Count > 1,
null, null);
this.SelectionData.ActionsPaneItems.Clear();
this.SelectionData.ActionsPaneItems.Add(new Microsoft.ManagementConsole.Action("Show Selected",
"Shows list of selected Users.", -
1, "ShowSelected"));
this.SelectionData.ActionsPaneItems.Add(new Microsoft.ManagementConsole.Action("Say Hello",
"Say Hello.", -1, "Say Hello"));
}
}
We have overriden OnSelectionChanged , this helps to customize the context menu on the action pane. Here we have added two menuitems and actions for those items.

// this defines what happens when an action is selected.
// Here, we have only one action and we invoke
// the ShowSelected method when it is selected.
protected override void OnSelectionAction
(Microsoft.ManagementConsole.Action action,
AsyncStatus status)
{
switch ((string)action.Tag)
{
case "ShowSelected":
{
ShowSelected();
break;
}
case "Say Hello":
{
MessageBox.Show("Hello, How are you?");
break;
}
}
}

///
/// Shows selected items.
///
private void ShowSelected()
{
MessageBox.Show("Selected Item: \n" + GetDetails());
}
private string GetDetails()
{
StringBuilder nodedetails = new StringBuilder();
foreach (ResultNode resultNode in this.SelectedNodes)
{
nodedetails.Append(resultNode.DisplayName + ": " +
resultNode.SubItemDisplayNames[0].ToString() + "\n");
}
return nodedetails.ToString();
}
When user select the ShowSelected menu item ShowSelected() functions gets called. This will show up a message box with the selected elements details.
This also allows multi selection.
Selection of menu item, Say Hello just pops up a message box. We can override the OnRefresh function as below.

protected override void OnRefresh(AsyncStatus status)
{
MessageBox.Show("Need to implement....");
}
Adding new child elements to the Root Node happens like this.

/// Initializes a new instance of the MMCListViewSnapInClass class.->Read More...
///
public MMCListViewSnapInClass()
{
// Create the root node.
this.RootNode = new ScopeNode();
this.RootNode.DisplayName = "My MMCListView";
// Create a message view for the root node.
MmcListViewDescription lvd = new MmcListViewDescription();
lvd.DisplayName = "My Special MMCListView";
lvd.ViewType = typeof(RootListView);
lvd.Options = MmcListViewOptions.ExcludeScopeNodes;
// Attach the view to the root node.
this.RootNode.ViewDescriptions.Add(lvd);
this.RootNode.ViewDescriptions.DefaultIndex = 0;
// Creating the child Nodes to the root Node
// Also specified names for each node
ScopeNode scopeOne = new ScopeNode();
scopeOne.DisplayName = "Gigy";
// Create a message view for the scopeOne.
MmcListViewDescription lvdOne = new MmcListViewDescription();
lvdOne.DisplayName = "Gigy's MMCListView";
lvdOne.ViewType = typeof(MyCusomtSelistView);
lvdOne.Options = MmcListViewOptions.ExcludeScopeNodes;
scopeOne.ViewDescriptions.Add(lvdOne);
scopeOne.ViewDescriptions.DefaultIndex = 0;
this.RootNode.Children.Add(scopeOne);
........
so on ......